Overview:
If you knew a female's name was Edna, how old would you guess she is? What about a male named Aiden?
In this assignment you will write a program to determine the average age of people with a given name. You will use two data sets from the Social Security office - the number of babies born each year with given names; and the probabilities that people are alive given their year of birth.
The Social Security Names Database:
Distributed with this assignment are a number of files with names dob.txt where is in the range 1900 to 2014 (inclusive). Each file contains comma-separated data in the form:
Name, Sex, Count
Thus, each line in the file shows the number of children born with a given name. Because some names are given to both girls and boys, the sex is also provided.
Social Security Mortality Tables:
The two other data files provided with the assignment are femaleProb.txt and maleProb.txt. Both files contain comma-separated data in the form:
Year, Probability
The probabilities indicate the likelihood that a person born in a given year is alive today. These values were computed from data the Social Security office collects on how many people die each year and their ages (Actuaries use this information to determine the cost of life insurance).
Combining the Datasets:
For a given name and year, these two datasets give us a way to estimate the number of people born with that name who are still alive. For example, There were 17611 boys named Anthony born in 1984, and there is a 0.961870072 probability that each of these individuals is alive today. Therefore, we would expect there to be 17611 * 0.961870072 = 16939.49 young adults named Anthony who were born in 1984 (We will truncate this number to 16939 for simplicity).
If we perform this computation for each year, we can create the following plot:
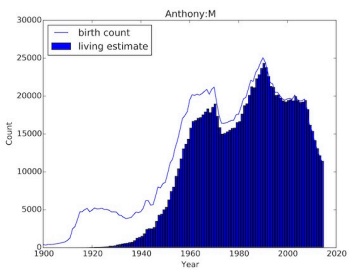
The line plot shows the number of boys named Anthony born each year, and the bars show the number of males named Anthony born each year that are probably alive today. As expected, anyone Anthony born in the very early 1900s is unlikely to be alive.
Specifications:
The file age_estimation.py contains a number of functions you must implement. The documentation contains specifications for what each function does, and the list below gives suggestions of how to write the code.
get_count(name, sex, year) - Your code should construct the filename from the year provide and then open that file. Read the file line-by-line until you find the proper line. Return the corresponding count or 0 if you read all lines without finding the proper match. Don't forget to close the file before you return!
compute_count_by_year(name, sex) - Starting with an empty list, call the get_count function with each year in the range [1900, 2014] and then append the return value to the list. When done, return the list.
load_probabilities(sex) - Use the parameter to determine whether you open femaleProb.txt or maleProb.txt. Once a file is open, start with an empty list, and read the file line-by-line to append the probabilities into the list.
When done, close the file and return the list.
product(list1, list2) - Iterate over both lists by index and append the product of the values to a new list. When done, return the new list.
compute_average_age(counts) - This function returns an estimate of the age of a person today. This can be done by computing the weighted average of the age of people using the counts as the weight. The parameter counts contains the number of people with the same name that is still alive today for each year since 1900. For each value of counts you can determine the current age of the people born each year (2016 minus the year when they were born). Keep a running sum of these products and then divide by the total number of people when done.
create_plot(name, sex, birth_counts, living_counts) - Create a plot similar to figure above. Be sure to add axis labels, a title, and a legend.
main script - Call the other functions: Load the probabilities and birth counts. Use these data sets to compute the living counts. Output the average age, and generate the plot.
As a reference, none of the functions in the solution implementation have more than 11 lines of executable Python.