Assignment Task:
The project: You are to build an "artificial" file system displayed by a graphical/text editor. The project has 3 parts: (1) creation of the file system; (2) feature extensions and maintainable structures; and (3) behavior of the file system.
The best solution is to consider and integrate Parts 1-3 altogether. Here, I split the project into 3 parts to help you solve this project step by step (or get partial credits). Your score will be lower if you cannot integrate them seamlessly together. Also, please note this is design patterns project, you should follow all good software design concepts and principles you've learned. Bad design will get points off.
Part 1: Creation of a file system:
You will implement the skeleton of a hierarchical file system using Builder, Singleton, and Composite patterns. Your file system should handle file structures of arbitrary size and complexity. It shouldn't put arbitrary limits on how wide or deep the file structure can get. From the implementer's perspective, the representation for the file structure should be easy to deal with and extend. (Note, please do not use File and Dir classes in Java/C#. These classes are to create real files/directories in your computer).
When your program starts, Builder pattern is in charge to parse a script that is composed by the commands as follows (script1.txt provided on Canvas). Builder pattern will then create a file system, using Composite and Singleton patterns, based on the script. For this project, do not worry about creating a very detailed and robust command line interpreter. You can assume that the user will always use the system in the proper way. At the start of the program, your user can assume that the file system is empty and they are in the "root".
mkdir - add a new sub-directory, called dirname, to the current
directory
create - create a new file in the current directory. Its name will be
filename, and the size attribute of the file will be the 2nd command line arg
cd | .. - this will change to a subdirectory named dirname, or
change to the parent directory (in the case of ".."); path expressions do not need to be supported
del | - this will delete the file named filename, or it will do a
cascading delete in the case of dirname
size | - this returns the size attribute of a filename; in the case of a
directory, it returns the size of ALL files and subdirectories ls | - in the case of filename, this will print the name of the file
and its size; in the case of dirname, it will list all of the files/sizes in the named directory; it is NOT cascading; if no parameter is given, default to current directory
Part 2: Feature extensions using maintainable structures
Create a new folder that stores the source code from Part 1. Revise the source code to make it maintainable.
1. Use the Visitor pattern to re-implement the "del" "size" and "ls" commands.
2. Use the Visitor pattern to implement "resize" command described as follows:
resize - resize an existing file in the current directory. Its name
will be filename, and the new size attribute of the file will be the 2nd command line arg
3. Use the Visitor pattern to implement "exit" command. It pretends to jump out of the file system. The "exit" will perform the action stated in (4). Don't forget to keep the instance of file system so user could access to it in Part 3.
4. Implement Proxy pattern for deleting files and directories. The "del" command will defer deleting a file or a directory until "exit" command is called.
Part 3: Behavior of a file system:
Revise the TreeDisplay concept covered in Adapter pattern (also shown as follows). (You may not need all the functions in TreeDisplay).
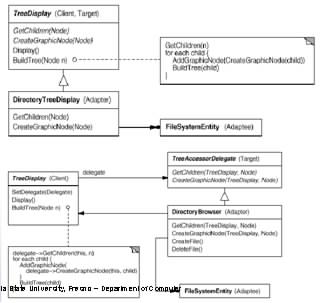
1. Implement Adapter pattern so that by calling display() function of DirectoryTreeDisplay the entire tree structure can be drawn. You only need to show the hierarchy of the file system, including names and file/dir sizes, in text format.
2. Introduce a header and footer to TextDisplay using Decorator pattern.
3. Implement Observer pattern to resize command. When "resize" is called, namely, when a file is resized, DirectoryTreeDisplay will display the new hierarchy of the file system with the sizes of directories and files. Note that you may need to run Builder again to see if it is working. script2.txt is available on Canvas for you to test.
Want to fetch higher academic grades in your Advanced Software Engineering based assignments and homework, without putting so much effort? Want to make a brilliant career ahead in this field? Then avail our Advanced Software Engineering Assignment Help service and ace your academic career!
Tags: Advanced Software Engineering Assignment Help, Advanced Software Engineering Homework Help, Advanced Software Engineering Coursework, Advanced Software Engineering Solved Assignments
Attachment:- Advanced Software Engineering.rar