Create a simple Web Application which collects gradings under a number of criteria, and then computes an aggregate mark. Without any styling applied, the input form needs to look like this:
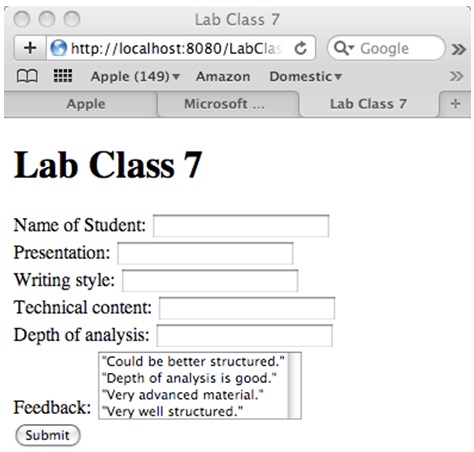
Presentation, Writing Style, Technical Content and Depth of Analysis must each be marked with a value between 0 and 100. Create above form.
Some additional constraints:
1. Use the same name, “score”, for each of the input fields which accepts a grade.
2. Permit multiple options to be chosen from drop down list.
3. Configure the form to submit a POST request when data is submitted, as we are working on the assumption that results would be “posted” into a database. Name like “AssessGrades” for the action which takes place when the form is submitted.
You now require to create a Servlet that would process Post request which is generated by the form submission.
Don’t forget to put it into a new packagewhen you create it.
Use the separate JavaBean to actually process grades into an aggregate mark. Though, you would require to extract raw data from the request message in the Servlet you have just created.
Extracting student name must be easy for you. But you require a little more information about the cases for data named “score” and the data from the Drop down list, where there copuld be multiple values under same name. What would happen here is that the form would associate the array of values with the respective name. You would use a slightly different method to get this array. Like:
String[] scores = request.getParameterValues("score");
So, start to work on the doPostmethod in your servlet now. You required to attain from the request object:
1. The name of the student;
2. The scores from each criterion;
3. The selected feedback statements.
You would also need to parse string representation of each score into a Double. Having got this far, you require to turn your attention to the JavaBean that would aggregate the scores into a single grade.
Create the new java class called (for example) Graderin a package called beans. This would have two attributes/fields: an array ofgrades; and a String that is the final_grade. Declare these.
You could now use NetBeans to create the “getter” and “setter” methods in a style which conforms to the JavaBeans standard. Right click in the editor and choose “Refactor/Encapsulate Fields”. You would not want a public “setter” method for final_grade, so deselect this. This is because the final grade is computed. Now your task is to compute this grade as follows.
At first, compute the mean of all the grades.
Then assess the final grade according to the following rules: Average ≥70 => “A”
60 ≤Average < 70 => “B”
50 ≤Average < 60 => “C”
40 ≤Average < 50 => “D” Average < 40 => “Fail”
You must now be able to use this Bean in the Servlet to get the overall final grade. Having calculated this, then create a response html document that reports back on the outcome. That report should include:
1. The name of the Student
2. The final grade
3. The feedback comments.
You can save a lot of network traffic and loading on the server side, if you check that valid data has been entered before the form is submitted. Include validation on the client side by adding some JavaScript into the index page to:
1. Check a name has been entered;
2. Check the value of each of the score fields is numerical and between 0 and 100.
Finally, once you have checked all the functionality is correct,use a bit of CSS magic to make both input and response forms look more attractive.