The Problem and Requirements
In this Project your task is to create a program that displays various levels of the fractal structure of the so called square shaped Sierpinski-carpet. The display of level 4 is shown below:
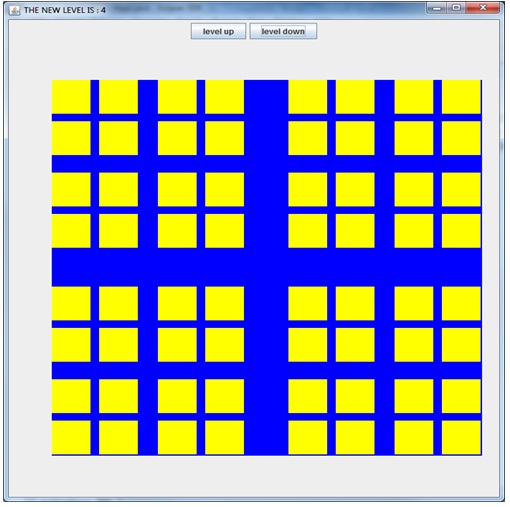
- GUI has two buttons to control level, one of them increases, the other decrease this parameter. The buttons are hosted by the panel at the NORTH region. Approximate fractal is shown at the CENTER region.
- To draw a given level all the smaller levels down to 1 must also be drawn, each four times on the divided smaller squares, hence a recursive drawing method must be implemented.
- Level 1 is just a yellow background square with no dividing blue strips. At level 2 the yellow square appears to be divided into four smaller squares by the vertical and a horizontal strip colored blue.
- At each step to the next level each yellow square is further divided by narrower blue strips.
- The size of the blue strips is always decreased by same proportion.
- Increase the level until yellow regions disappear and take note of the highest level at which yellow is still visible.
Analysis and Design
You have to define two classes: Fractal and Application for this program.
Fractal
- This class is responsible for the GUI (the frame and the graphics) and for running the recursive drawFractal( ) method.
- Fractal extends JFrame and implements ActionListener.
- Fractal has an inner class Centerthat extends JPanel. It represents the center panel and the graphics on it. The innerclass implements the paint method which in turn calls the drawFractal( ) method.
- Data fields (suggested) :
i) num, denom; publicintegers for the numerator and denominator of the shrinkage ratio
ii) point; a Point reference variable. Point is class in java.awt with two public int fields x and y. Point objects make it easier to reference points on the screen. Being public, direct references point.x and point.y are available for the coordinates; point always identifies the upper left corner of the current (sub)square in the fractal
iii) width, hight; int variables for the dimensions of the current square in the fractal (NOT the dimensions of the frame)
iv) level; int to store the fractal level
v) up, down; JButtons
i) changeFractal( ) ; sets the title of the whole frame showing the current level; instantiates a Center object (inner class) and adds the object to the pane; calls setVisible( )
ii) actionPerformed( ); mandatory for the buttons; adjusts the level value according to the button clicks and calls changeFractal( )
iii) makeWindow( ) builds and displays frame as shown on a template (without the colored fractal); registers the buttons; use 700, 800 when you set the size
iv) drawFractal( ); takes five parameters as follows: a Graphics reference, a Point reference, integers for level, width and height; the method needs three local variables for Point references: top, side, inside. These reference the upper left corners of the three new smaller squares at the upper right, lower left and lower right positions. Having the three new points and the width and length values determined according to the shrinkage ratio, the method calls itself four times using the four new sets of parameters. Note that the upper left corner of the upper left square is the same as the point reference of the caller method. Do not forget to handle the base case and the coloring
v) Constructor; takes four parameters to initialize the Point object, width, length and level; calls the makeWindow( ) method
Application:
This class has the main method where you:
-
declare and instantiate a Fractal object; initialize the fields with the parameters new Point(60,60), 600, 600, 0
- initializenum and denom to 9 and 20.
Documentation and Style:
The program should conform with the Java Documentation and Style Requirements as set by the Computer Science Department. Emphasis will be placed on having the required banner and internal comments, indentation, and overall professional appearance. Comments must be clearly written with correct grammar and spelling.