Overview
This assignment models a simplified delivery company. It is composed of the following departments:
i) receiving that contains a list of packages to be delivered,
ii) shipping which ships the packages
iii) accounting which monitors the packages delivered, packages shipped, how they are shipped, where they are delivered to and revenues.
The project simulates processing of a given set of packages. The packages consist of either standard delivery packages or overnight packages. The vehicles consist of planes and trucks. The accounting department records revenues and shipments.
Class Design
The application should include classes in the class diagram below. All data members should be private.
Note: This class diagram does not include all of the methods and data members required to implement this design.
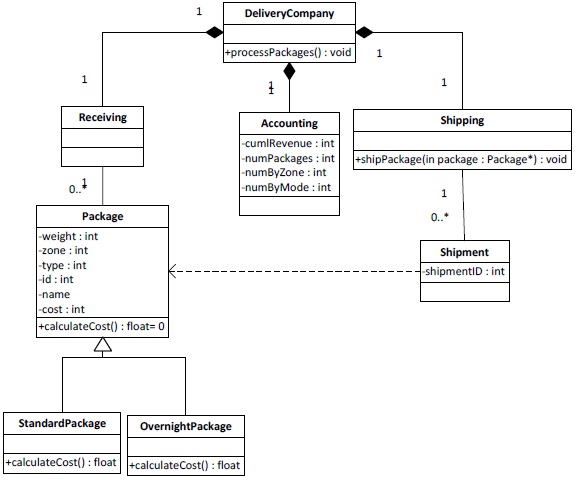
Class Descriptions
DeliveryCompany
The delivery facility consists of Receiving, Accounting and Shipping Departments. It has function, processPackages(), that processes the packages from Receiving to Shipping and conveys information to accounting.
Receiving
Receiving Department consists of a list of packages to be delivered. It makes available the information on each package. The transport mode, truck or plane, is estimated using a lookup table based on the zone the package is to be delivered to.
Shipping
Shipping Department ships packages by creating a shipment object for each package. It allots a shipping ID to each package.
Accounting
Accounting Department tabulates number of packages shipped by zone and mode of transport and the total revenue.
Package Class Hierarchy
The package class hierarchy consists of base class Package and two derived classes: StandardPackage and OvernightPackage. Base class contains an ID, addressee name, zone to be mailed to, weight, and a cost parameter. The derived classes calculate costs based on the cost parameter stored in the base class. The overnight package rate is a flat fee as a function of the zone and the standard package rate is a function of the weight and the zone that is the rate per pound would depend on zone the package is being sent to.
Assignment Requirements
Implement delivery company using the classes specified in the class diagram above.
Construct cpp file named DeliveryRun.cpp with main() in it. That main would have two statements: one would instantiate a DeliveryCompany object and the other would call the method processPackages() using that object.
The packages to be delivered are contained in ShippingCompanyInput.txt. Each line in the file represents a package and contains the following information:
• Priority (O = overnight and S = standard)
• Name of customer (no spaces in the customer name)
• Zone to be delivered to (1, 2, or 3)
• Weight of the package (in pounds)
The charges for each type of package to each zone are included in the Util.h file. The overnight rate is a function of the three delivery zones (OVERNIGHT_RATE). The standard rates are given per pound for each of the delivery zones (STANDARD_RATE_PER_POUND).
The transport mode for each package is a function of the type of package (overnight or standard) and the delivery zone (1, 2, or 3). These are given in OVERNIGHT_MODE and STANDARD_MODE in util.h.
The output must consist of:
• A detailed listing of each package
• A detailed listing of each shipment
• A summary of the shipments - Number of packages
• By transport mode (plane or truck)
• By zone (1, 2, or 3)
• Total - Total revenue