Assignment:
The TestCircle class is a Java application that creates a Circle object using the class, Circle, which incorporates the class, Shape. The TestCircle class displays the name of the shape; the radius as an X, Y coordinate location; and the area. It also changes the radius to a new value and then displays the same information in a different format. The class should not be able to create an object of type shape.
The source code for the TestCircle, Circle, and Shape classes have several syntax, semantic, and logic errors. Perform the following steps to debug the classes.
1. Read through the code of TestCircle, Circle, and Shape and fix any errors that you see.
2. Save the files.
3. Compile the classes. If complier displays any compilation errors, fix the errors and recompile.
4. When you've fixed all syntax and semantic errors so that classes compile without errors, run the TestCircle program and look for runtime and logic errors. Fix all errors and recompile.
5. When the program runs clean it should produce the output shownimport java.text.DecimalFormat;
public class TestCircle
{
public static void main(String[] args)
{
DecimalFormat showTwoDecimals = new DecimalFormat( "0.00" );
Circle c1 = new Circle(2.5, 22, 44);
System.out.println("This shape is a " + c1.getName() +
"\nlocated at " + c1.getLocation() +
"\nRadius is " + c1.getRadius());
System.out.println("The shape area is: " + showTwoDecimals.format(c1.area())+"\n");
c1.setRadius(4.25);
System.out.println("\nThe changed shape is:\n" +
c1 + "\nArea is " + showTwoDecimals.format(c1.area())+"\n");
}
}
public class Circle extends Shape
{
private int x=0, y=0; // coordinates of the center
private double radius;
// Constructors
public Circle()
{
setRadius(0);
}
public Circle(double r, int x, int y)
{
x = x;
y = y;
setRadius(r);
}
// Get radius of Circle
public double getRadius()
{
return radius;
}
// Set radius of Circle
public void setRadius(double r)
{
radius = ( r >= 0 ? r : 0 );
}
// Calculate area of Circle
public double area()
{
return Math.PI * radius * radius;
}
// convert the Circle to a String
public String toString()
{
return "Circle with center at (" + x + ", " + y + ")" +
" and radius = " + radius;
}
// return the class name
public String getName()
{
return "Circle";
}
// return the center location
public String getLocation()
{
return "X = " + X + ", Y = " + Y;
}
}public class Shape
{
public double area()
{
return 0.0;
}
public double perimeter()
{
return 0.0;
}
public String getName();
public String getLocation();
}
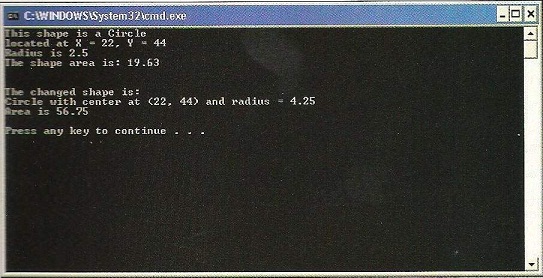