Problem
Sorting is a frequent array operation. An array can be sorted if there is an ordering relation between the elements in array. For illustration, if the array comprises a set of integer elements, we can order the elements according to integer number line. A common technique whereby the elements in an array might be sorted is called the bubble sort. Supposing an array of positive integers which we wish to sort according to the sequence represented by integer number line, the bubble sort operates as follows:
1. Find out the two adjacent elements, X and Y, in the array such that X > Y and swap X and Y, then sort the resulting array.
2. If there is no adjacent pair of the elements, X and Y, in the array such that X > Y, the list is sorted.
Note that the reason of swapping two elements X and Y that occur out of order is so that after the swap, the new list is closer to a sorted list. After a sufficient amount of swapping, we must end up with all the elements in order. For illustration given the array:
{1 2 5 4 7 3 6 8 10 9}
We would commence by the finding elements 5 and 4 and swap them to get:
{1 2 4 5 7 3 6 8 10 9}
We would then continue as follows:
{1 2 4 5 7 3 6 8 10 9}
{1 2 4 5 3 7 6 8 10 9}
{1 2 4 3 5 7 6 8 10 9}
{1 2 3 4 5 7 6 8 10 9}
{1 2 3 4 5 6 7 8 10 9}
{1 2 3 4 5 6 7 8 9 10}
The procedure is known as a bubble sort since elements slowly bubble up to their correct location.
Implement and Design a Java program which sorts a 10 element integer array using bubble sort procedure. The elements of the array to be sorted must be supplied by the user (assume the user will not input duplicates).
Make a GUI front end for your bubble sort program. The result must look something like that presented in Figure. You might use any graphic element to make your display - JOptionPanesare the easiest and most basic tools accessible to you, but you might also experiment with additional GUI controls such as JFrames, JPanels, etc. I would advise you to use objects from the Swing library (those objects begin with 'J') as opposed to objects from AWT library - Swing objects are a little easier to use and are ultimately more flexible and robust than AWT objects.
In the illustration below, there are 10 text fields on a JFrame background to allow input of array elements (remember that for each text field you should press the carriage return key to invoke the listener). When the array has been populated, we press the start button, at which point the given array is output as a label. We then sort the output and result the sorted array.
Hint: Don’t attempt to make the GUI too sophisticated. Just concentrate on producing a working result (although sound programming techniques should still be applied).
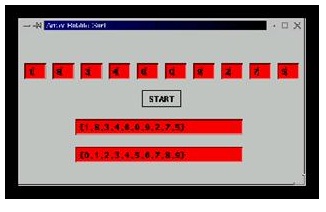
Figure: Bubble sort GUI (partial example)
Remember to write the source code for each class in a separate file which should have the same name as the class name together with extension .java. Remember also that by convention, class names commence with a capital letter.