The purpose of this assignment is to get you started with C++ programming. You'll develop simple programs (with input and output) to solve simple mathematical and engineering problems.
1. Write a program to compute the area A of any ellipse with semiaxes a and b. Prompt the user to enter the lengths a and b. Set the precision of the area to two decimal points. (The area of an ellipse is computed using this formula: Area= π a∗ b.)
Sample Output:
Enter the length of the semiaxes: 12 15
The area of an ellipse with semiaxes 12 and 15 is 565.49
2. Consider the following circuit.
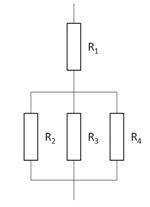
Ohm's Law describes the relationship between voltage (V), current (I) and resistance (R). To calculate total resistance for multiple resistances in series, the following series resistance formula is applied:
RTotal = R1 +R2 +. . .+Rn
where R is each individual resistor. To calculate the total parallel resistance, the following parallel resistance formula is applied:
RTotal = 1/ (1/R1)+(1/R2)+. . .+(1/Rn)
where R is each individual resistor. Write a program that reads the resistances of the four resistors and computes the total resistance, using Ohm's law. Set the precision of the final total resistance to two decimal points. HINT: To measure the total resistance of this circuit, you need to calculate the total parallel resistance of R2, R3, and R4 first. Then, calculate the total series resistance of R1 and the calculated total parallel resistance.
Sample Output:
Enter the value of R1: 10
Enter the value of R2: 20
Enter the value of R3: 20
Enter the value of R4: 20
The total resistance for this circuit is: 16.67 ohms.
3. The circuit shown below illustrates some important aspects of the connection between a power company and one of its customers. The customer is represented by three parameters, Vt, P, and pf. Vt
is the voltage accessed by plugging into a wall outlet. Customers depend on having a dependable value of Vt in order for their appliances to work properly. Accordingly, the power company regulates the value of Vt carefully. P describes the amount of power used by the customer and is the primary factor in determining the customer's electric bill. The power factor, pf, is less familiar. (The power factor is calculated as the cosine of an angle so that its value will always be between zero and one.) In this problem you will be asked to write a C++ program to investigate the significance of the power factor.
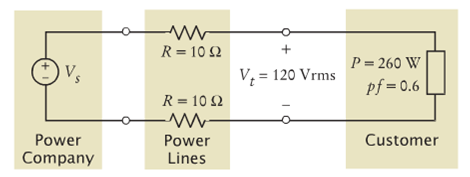
In the figure, the power lines are represented, somewhat simplistically, as resistances in Ohms. The power company is represented as an AC voltage source. The source voltage, Vs, required to provide the customer with power P at voltage Vt can be determined using the formula
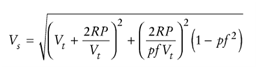
(Vs has units of Vrms.) This formula indicates that the value of Vs depends on the value of pf. Write a C++ program that prompts the user for a power factor value and then prints a message giving the corresponding value of Vs, using the values for P, R, and Vt shown in the figure above. Set the precision of Vs to two decimal points.
Sample Output:
Enter the power factor, pf: 0.75
P = 260 W, R = 10 Ohms, and Vt = 120 Vrms
Vs = 167.75 Vrms