Homework: Simple 5-Card Poker Game
In this assignment, you will develop a simple poker game, complete with basic AI, using the object oriented programming principles.
UML Class Diagram:
Your assignment must contain and use the following classes:
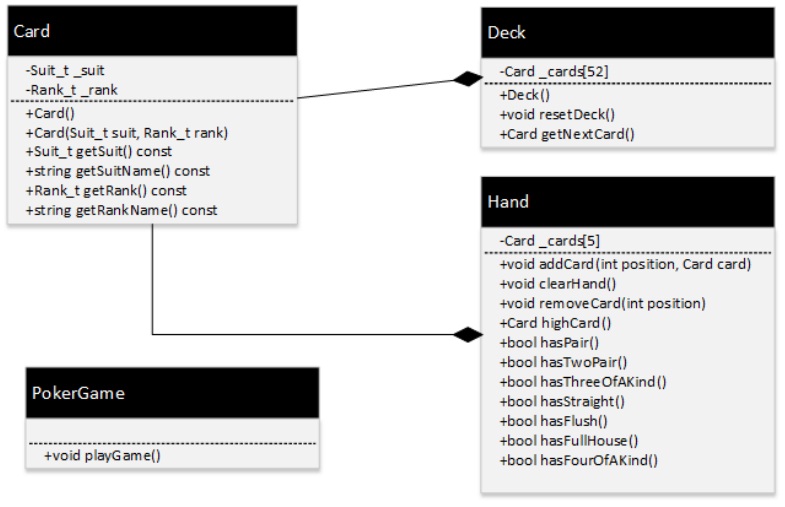
Card and Deck Classes:
The Card and Deck classes are direct carryovers from Lab #2. For more information about these classes, see the Lab #2 document on the course website.
Hand Class:
You will need two instances of the Hand Class in your game: one for the player and one for the computer. Each Hand will contain the respective player's current hand of cards. The hand class must have several helper methods that can be used to determine the strength of the hand. If you are unfamiliar with poker hands, Wikipedia has a related article that may be of interest.
PokerGame:
Like the BlackJack class in Lab #2, the PokerGame class will be used to encapsulate all of the logic associated with playing the game of poker. The play method's operation will be discussed in the next section.
Flow of Play:
Your poker game's play() method should operate as follows:
A) Deal the player five cards and display the results on the screen
B) Ask the player which cards he or she would like to exchange
C) Remove the desired cards from the player's hand
D) Deal the player N new cards (N = exchange amount)
E) Display the new hand on the screen
F) Deal the computer five cards and display the results on the screen
G) The computer determines how many cards to exchange based on the following logic:
• Zero cards if the computer has a four of a kind, full house, straight, or flush
• One card if the computer has two pair
• Two cards if the computer has three of a kind
• Three cards if the computer has a pair
• Five cards if the computer has none of the above
H) Remove the appropriate number of cards in the computer's hand
I) Deal the computer the appropriate number of replacement cards
J) Display the new hand on the screen
K) Determine the winner of the game based on the following rankings:
• Four of a kind
• Full house
• Flush
• Straight
• Three of a kind
• Two Pair
• Pair
• High Card (ordered by Spade, Heart, Diamond, with Club being the lowest)
L) Indicate the winner on the screen
Sample Output:
*** Simple 5-Card Poker ***
The cards have been shuffled and you are dealt:
1. Five of Hearts
2. Five of Spades
3. Jack of Hearts
4. Two of Clubs
5. Ace of Diamonds
Indicate the cards that you would like to exchange (-1 to end):
4
3
6
Invalid selection.
Indicate the cards that you would like to exchange (-1 to end):
-1
Your new hand is:
1. Five of Hearts
2. Five of Spades
3. Two of Clubs
4. King of Hearts
5. Ace of Diamonds
The computer is dealt the following cards:
1. Ace of Spades
2. Three of Spades
3. Five of Diamonds
4. Ace of Hearts
5. Six of Clubs
The computer exchanges cards 2, 3 and 5. The new hand is:
1. Ace of Spades
2. Seven of Clubs
3. Seven of Diamonds
4. Ace of Hearts
5. Queen of Diamonds
You have one pair.
The computer has two pair.
The computer wins.
Header Comment and Formatting:
1. Be sure to modify the file header comment at the top of your script to indicate your name, student ID, completion time, and the names of any individuals that you collaborated with on the assignment.
2. Remember to follow the basic coding style guide.
Required Game Play Components:
a) The program randomly deals cards to both the player and the computer.
b) The program prompts the user for cards to exchange.
c) The program correctly identifies and handles invalid input during the card exchange phase.
d) The program deals the requested number of cards to the player after the exchange phase.
e) The computer selects the number of cards to redraw based on the algorithm outlined in the "flow of play" section.
f) The program correctly determines the winner of a given game.