Your Task:
“Days-to-Go” is an interesting and useful feature in website design. It shows up the remaining number of days (or even with hours, minutes, and seconds) towards an event. For example, at anytime you visit the official website of Rio 2016 Olympic Game1, you will see such a feature showing the number of days, hours, minutes and seconds towards the starting date of Rio 2016. In this assignment, you are going to write a JavaScript program to implement the “Days-To-Go” feature.
The Concept of Time in JavaScript:
In JavaScript a time is defined as a Date Object. Each date object stores its state as a time value, which is a primitive number that encodes a date as milliseconds since 1 January 1970 00:00:00 UTC. Thus, a date later than 1 January 1970 00:00:00 UTC will have a positive time value, whereas an earlier date will have a negative time value. On the basis of the common timeline (which we all live
on), the distance between any two dates can be calculated using their time values in milliseconds. Figure illustrates the concept, where C is a date earlier than 1 January 1970 00:00:00 UTC, and A and B are later with B being further than A.
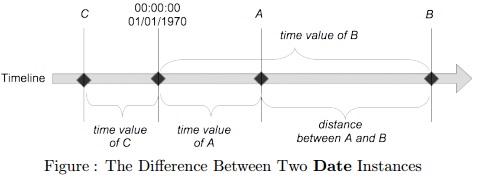
Functional Requirements:
Constants and Variables:
Within the script section, create constants and variables following professional conventions and initialise them using right values. Some constants and variables have been suggested in the following tables. You should create more when necessary.
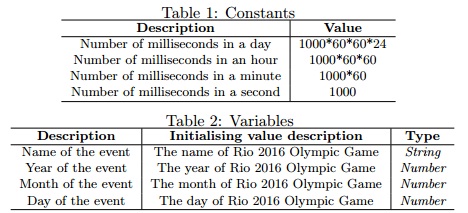
Calculation:
1. Create a Date object for the date of the event by using the variables created previously.
• Send the object constructor the variables (not values) for year, month, and day of the event;
• Mind the order of arguments sent to the constructor;
• Note that month numbers begin at 0 for January, 1 for February, and so on;
2. Create a Date object for the current time.
• No arguments need to be supplied to the constructor.
3. Calculate the difference between the current time and the event time:
• Use the getTime() member function to get a Date object’s time value in milliseconds
• Deduct the time value of current time by using the value of event time.
4. Calculate the number of days to the event:
• Divide the time value difference by the number of milliseconds in a day.
• Use the Math.floor() function to reduce the result number to an integer.
5. Calculate the number of hours, minutes, and seconds in the remaining time value:
(a) Mod the time value difference by the number of milliseconds in a day;
(b) Divide the mod result by the number of milliseconds in an hour;
(c) Use the Math.floor() function to reduce the number to an integer for the number of hours;
(d) Repeat Steps (a) to (c) to calculate the number of minutes and seconds. You may need to update the calculating formula accordingly.
Presentation:
Figure shows a sample output when running the “DaysTo-Go” program. Note that
- the information should be displayed using the alert() function;
- wherever possible you should use variables in expressions instead of explicit values (e.g., literals and numbers), for example, using the variable created for the event name instead of a string value of “RIO 2016”;
- the layout of output may vary depending on web browsers.
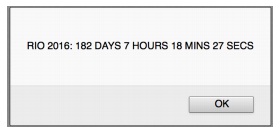
Non-functional requirements:
Structure of the Source Code:
- All code should appear in the script section in the head of the HTML document.
- Do not write any code in the HTML body. All functionality are delivered by JavaScript.
- In the script order your code as follows:
(a) Constants;
(b) Variables and objects (declared and initialised);
(c) Other statements.
Comments:
- You are required to add at least three comments to the source code.
- Do not comment every single line, instead, comment on blocks of code with a common purpose.
- Do not simply translate the syntax into English for comments, instead, describe the purpose of blocks of code.
Submission:
What You Need to Submit – Two Files
For a complete submission you need to submit two files as specified below. You can submit them individually or compress them and submit a common .zip (or .rar ) file. The assignment submission system will accept only the files with extensions specified in this section.
1. Statement of Completeness in a file saved in .pdf format in 200-300 of your own words describes:
- The state of your assignment, such as, any known functionality that has not been implemented, etc. (It is expected that most people will implement all of the functionality of this assignment.)
- Problems encountered, such as, any problems that you encountered during the assignment work and how you dealt with them;
- Reflection, such as, any lessons learnt in doing the assignment and suggestions to future programming work.
2. The program in a file saved with an .html extension contains the source code implemented following the functional and non-functional requirements.