Question1)a) A function is defined on [0,∞) as follows:
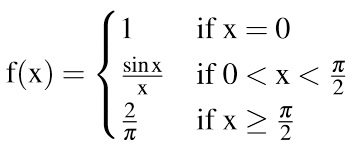
Write a C function to calculate this function in the interval [0,M) where M is the maximum possible long double number. You can use an approximation for p in your function.
b) A life-insurance saleswoman earns commission for selling policies as follows:
• If the policy amount is ≤ Rs. 15,000, the commission is 0.4% of policy amount.
• If policy amount is ≥ Rs. 15,000 but less than or equal to Rs. 30,000, the commission is Rs. 60+0.6% of the amount in excess of Rs. 15,000.
• If the policy amount is more than Rs. 30,000, the commission is Rs. 150 +0.8% of the amount in excess of Rs. 30,000.
Write a C program that reads the amount of insurance sold and outputs the commission of the saleswoman.
Question2)a) What does the following program do? Explain, line by line, starting from line 5, how it works.
1 #include
2 int main()
3 {
4 int i, k;
5 char * string = "To be or not to be.";
6 for (i = 1; * ++string;)
7 i++;
8 for (k = 1;k <= i; k++)
9 printf("%c",string[-k]);
10 return 0;
11 }
b) Write a program that does the following:
i) Declares a struct that stores the name and age of a person.
ii) Uses typedef to name the struct as data.
iii) Declares a variable of type data , read the details of a person from the terminal using scanf() and stores it in the variable declared.
iv) Prints whether the age of the person is above 40 or below 40 using a function that takes data as input and prints appropriate output.
Question3)a) The following program reads an unsigned long integer and prints its binary expansion. Explain, line by line, starting from line 5, how it works.( Note: This program is written for a machine in which unsigned long is 4 bytes long. Also, you may have to append ‘L’ to the number you are entering.)
1 #include
2 int main()
3 {
4 unsigned long int i, j=1;
5 int k,a[33];
6 printf("Enter a natural number:\n");
7 scanf("%lu\n",&i);
8 for (k = 0; k<= 32;j*= 2,k++)
9 a[k]=i&j?1:0;
10 while (a[--k]==0);
11 for (;k >=0;k--)
12 printf("%d",a[k]);
13 return 0;
14 }
b) What does the following function do? Explain, line by line, how it works.
1 int function(unsigned long i)
2 {
3 int k=1;
4 while (i>>=1){
5 k++;
6 }
7 return k;
8 }
Question4)a) How will you represent the following array as a sparse array using vector representation?
0 0 3 0 0 1 0
0 0 0 0 1 0 3
3 0 0 0 0 1 1
0 0 0 0 3 0 0
0 2 0 0 0 0 0
0 0 0 0 4 0 0
0 0 0 0 2 0 0
Question5) Write a C program that creates a binary search tree and add the numbers 12, 3,0,7,8 and 9 to the tree. Your program should have a function to add nodes to a binary search tree.